Understanding Python's MutableSet A Guide to Resolving AttributeError in Collections Module
Understanding Python's MutableSet A Guide to Resolving AttributeError in Collections Module - Introduction to Python's MutableSet and Its Role in Collections
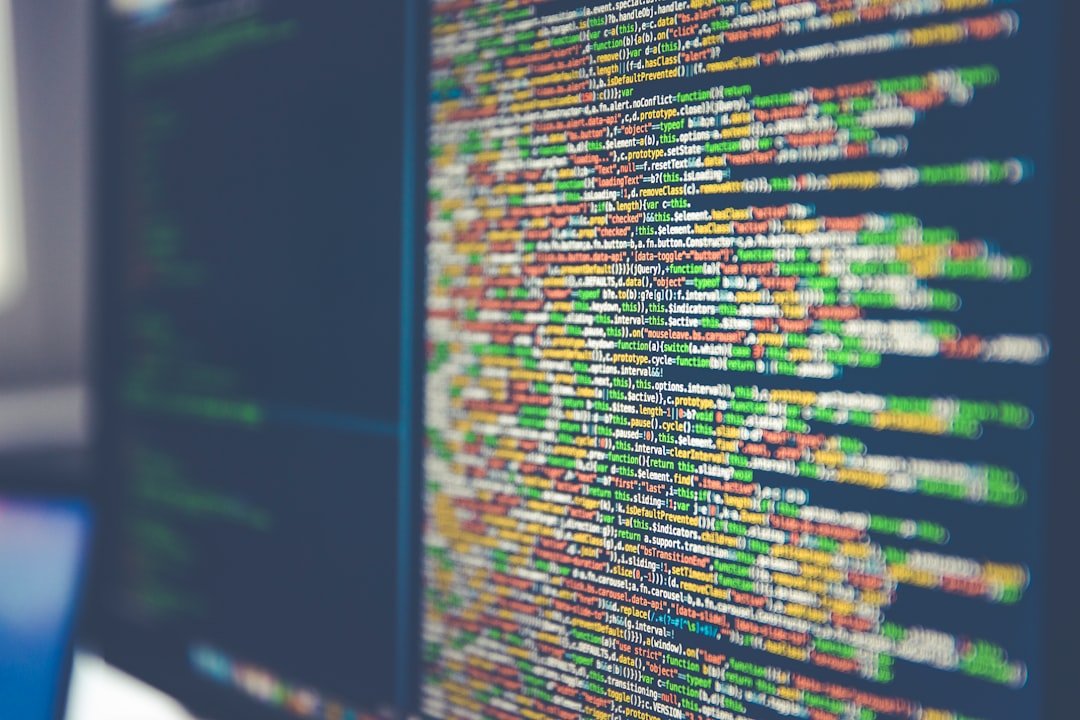
Python's `collections` module offers various data structures beyond the standard list, tuple, dictionary, and set. One such structure is the `MutableSet`, which, as its name suggests, allows you to modify the set after it has been created. This flexibility is particularly useful for dynamic scenarios where the elements of the set might change over time.
However, with Python 3.10 and later, the `collections.abc` module became the home for `MutableSet`. This move is important because using the old import statement from `collections` will result in an `AttributeError`—a reminder that Python evolves, and keeping up with these changes is critical for smooth development.
The `MutableSet` is just one piece of the puzzle within the `collections` module. Other specialized types such as `Counter`, `deque`, `defaultdict`, and `OrderedDict` cater to distinct data handling requirements. Familiarizing yourself with these specialized collections can often lead to cleaner and more efficient code in your Python programs.
The `MutableSet` class is an abstract base class, meaning it can't be used directly to create objects. Instead, it acts as a blueprint for building mutable sets. This allows for the creation of more customizable collections that can be tailored to specific needs. It's a bit like a framework for a house – you can't live in it directly, but it provides a structure for you to build your own customized home.
The `MutableSet` class lets developers define how their collections manage membership and duplicate rules, a capability not found in the standard Python `set`. But this flexibility comes with a cost – the `remove()` method throws a `KeyError` if the item is not found, making it critical to handle this exception properly within your code. When subclassing `MutableSet`, you must implement all of its required methods, ensuring that your custom collection conforms to expected set behaviors.
`MutableSet` can be used as a foundation for creating specialized data structures that merge set features with other collections. However, the performance of these methods depends on the implementation. Failing to consider the time complexity of these methods when working with large datasets can lead to inefficiency and unexpected performance bottlenecks.
Custom hash functions can be used with `MutableSet`, which can improve integration with other Python data structures that rely on hashability. By defining a consistent interface, `MutableSet` helps to avoid the `AttributeError` encountered when using methods not implemented for standard sets.
While `MutableSet` allows for custom object creation, it's important to understand Python's underlying data models to effectively manage memory and performance. Creating a custom `MutableSet` can be beneficial when built-in types don't meet your needs, allowing you to tailor collections and optimize existing processes.
Understanding Python's MutableSet A Guide to Resolving AttributeError in Collections Module - The Shift from collections to collections.abc in Python 10
In Python 3.10 and beyond, a critical change took place with the move of abstract base classes like `MutableSet` from the `collections` module to `collections.abc`. This shift underscores the importance of staying current with Python's evolution, as using older import methods from `collections` will now result in `AttributeError`. This transition highlights a key strength of Python 3.10 and later - the `collections.abc` module empowers developers to build custom containers by providing a solid foundation through its abstract base classes. These classes act as templates, ensuring that custom collections conform to specific interfaces. This focus on interface adherence strengthens code consistency and reduces errors. This move represents a deliberate effort by the Python developers to improve code structure and clarity. As Python's landscape continues to evolve, understanding these changes is crucial for developers to ensure compatibility and take advantage of Python's growing data handling capabilities.
The shift of `MutableSet` from `collections` to `collections.abc` in Python 3.10 isn't just about a simple location change. It reflects a broader shift in Python's design, emphasizing a clear separation between concrete collections and abstract base classes. This separation promotes cleaner code and easier understanding of object-oriented design principles.
While Python 3.10 and later still allow the old import statement (`collections.MutableSet`), developers are encouraged to adapt to the new `collections.abc` path. This allows developers to leverage the flexibility of custom `MutableSet` implementations with additional support for methods like `__ior__`, making set operations more intuitive.
This move also lets developers go beyond the standard set behaviors by defining custom rules for membership in `MutableSet` subclasses. This opens doors for creating highly specialized and innovative data structures tailored for particular use cases.
The integration of `MutableSet` with other abstract base classes in `collections.abc`, such as `Iterable` and `Sized`, adds another layer of flexibility to custom collections. This makes it easier to combine functionalities, leading to more robust data structures.
The introduction of generic type support in Python 3.10, which allows developers to specify the type of elements stored in `MutableSet` using type annotations, is a welcome addition. This feature improves type checking during development, potentially preventing runtime errors and making code more reliable.
However, the transition also brings about performance considerations. While `MutableSet` from `collections.abc` offers enhanced functionalities, developers must carefully consider the time complexity of their implementations, particularly when dealing with large datasets, to prevent potential performance bottlenecks.
The new framework unlocks more complex functionalities in `MutableSet`, such as chainable methods similar to functional programming styles. These advancements provide developers with more expressive tools for data manipulation.
It's important to remember that custom implementations of `MutableSet` require explicit error handling, as demonstrated by the `KeyError` thrown by `remove()` if the item isn't found. This is a crucial difference from standard sets, where error handling might be less explicitly handled.
In conclusion, the transition of `MutableSet` to `collections.abc` presents a significant change in Python's data structure landscape. While it introduces new opportunities for creating flexible and powerful custom collections, developers must be mindful of the nuances, including the need for careful error handling and potential performance challenges, to effectively leverage this powerful tool.
Understanding Python's MutableSet A Guide to Resolving AttributeError in Collections Module - Common Causes of AttributeError with MutableSet
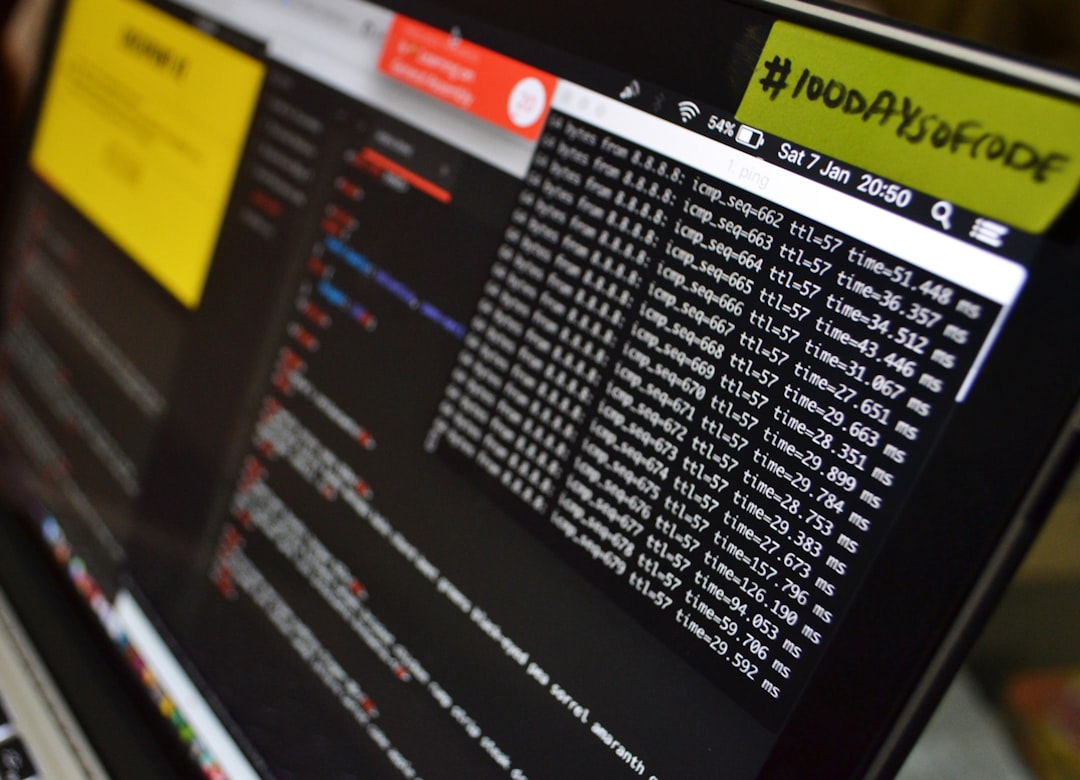
The `AttributeError` you encounter with Python's `MutableSet` usually arises because of its relocation to the `collections.abc` module, beginning with Python 3.3. If you're still importing `MutableSet` from the outdated `collections` module in versions 3.10 or later, you'll run into this error. To fix this, update your import statement to `from collections.abc import MutableSet`. Other common causes for `AttributeError` include outdated import references in legacy packages or compatibility issues stemming from discrepancies in your Python version. Therefore, it's crucial to stay diligent about your import paths and maintain compatibility with your Python version to avoid these problems when working with mutable sets. Additionally, you should be mindful of package-specific issues, particularly within third-party libraries that might not have been updated, as these can also contribute to errors.
The `AttributeError` when working with Python's `MutableSet` is more common than one might initially think, especially in Python versions 3.10 and beyond. This error can arise due to several reasons, highlighting the importance of understanding the underlying mechanics of `MutableSet`.
One common reason for the error is failing to implement all required abstract methods when subclassing `MutableSet`. This omission, a simple oversight for developers new to Python's class system, leads to an immediate `AttributeError` when trying to instantiate the subclass.
Another pitfall is importing `MutableSet` from the `collections` module instead of `collections.abc`, a practice that is incorrect in Python 3.10 and later. This misdirection results in an `AttributeError`, a stark reminder to keep up with Python's ever-evolving landscape.
The dynamic nature of Python's objects can also contribute to `AttributeError` when working with `MutableSet`. As Python allows for the creation of attributes at runtime, unintended attributes can clash with the `MutableSet` structure, causing confusion.
Furthermore, developers must ensure proper method chaining when overriding abstract methods from `MutableSet`. Not calling the superclass method can lead to unforeseen `AttributeError` upon invoking certain operations, demanding careful consideration of method inheritance.
Providing custom objects as elements within a `MutableSet` can add another layer of complexity, particularly when these objects lack proper `__hash__` and `__eq__` implementations. This missing setup is often responsible for `AttributeError` during operations involving custom objects within the set.
It is crucial to maintain consistent data types within a `MutableSet`. Attempting to use non-hashable types, such as lists or dictionaries, can disrupt expected behavior and trigger `AttributeError`. This underscores the importance of understanding data type constraints when working with specialized structures.
Version incompatibility, especially when using older Python versions, is a frequent culprit of `AttributeError` with `MutableSet`. This error emphasizes the need for developers to be mindful of the evolution of Python's features and the necessary updates in their code to maintain compatibility.
Circular dependencies, when elements within a `MutableSet` are interrelated in a cyclical manner, can lead to unpredictable behavior, often manifesting as `AttributeError`. Careful design to avoid this complex interplay is critical to ensure proper functionality.
Developers might accidentally create `MutableSet` instances with incorrect constructor parameters, leading to silent failures and eventual `AttributeError`. This underscores the need for strict parameter validation to prevent unintended misuse of the class.
Last but not least, a misconfigured Python environment or inconsistencies between dependencies can contribute to `AttributeError` related to `MutableSet`. Maintaining a well-defined and consistent environment is essential for successful code execution.
Understanding these potential causes of `AttributeError` is essential for developers working with `MutableSet` in Python. While `MutableSet` offers significant flexibility in creating customized data structures, its effective utilization demands careful attention to details and adherence to its underlying rules.
Understanding Python's MutableSet A Guide to Resolving AttributeError in Collections Module - Correct Import Syntax for MutableSet in Modern Python Versions
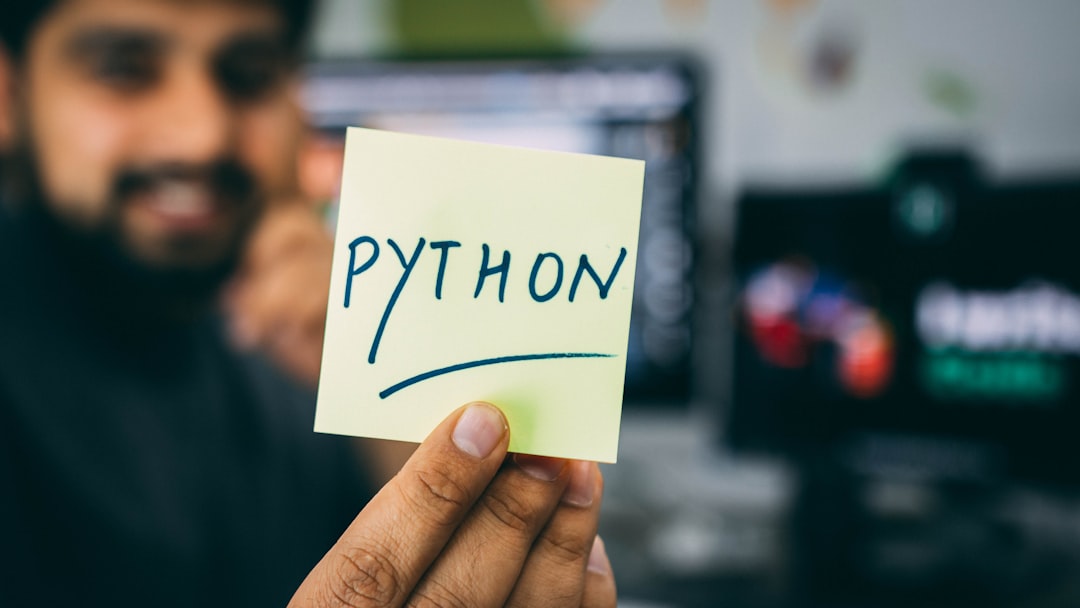
In modern Python versions, specifically since Python 3.3, `MutableSet` has moved from the `collections` module to `collections.abc`. This means the import syntax you use needs to be updated to avoid errors. You must use `from collections.abc import MutableSet` instead of the outdated `from collections import MutableSet`. If you're using an older version of Python (before 3.3), then the original import statement should work as intended. Staying up-to-date with these changes is important for developers to avoid issues and take advantage of Python's evolving features.
Python's evolution continues, and one noticeable change involves the `MutableSet` class. While in earlier versions, you'd import it from `collections`, in Python 3.10 and later, the correct path is `collections.abc`. This move reflects Python's commitment to clearer design and interface compliance, ensuring that your custom `MutableSet` implementations adhere to expected behaviors. Although older code might still function, sticking to the new import path keeps things organized and prevents confusion.
This shift emphasizes a crucial aspect of working with `MutableSet` — the need for proper error handling. Since `remove()` throws a `KeyError` if the element doesn't exist, you must carefully implement exception handling, unlike standard sets which might handle errors more subtly.
The introduction of generics in Python 3.10 adds another layer to `MutableSet`. Specifying the type of elements stored in the set, via type annotations, can help catch errors early and make your code more reliable. However, remember that performance is crucial, especially when customizing `MutableSet`. You need to be mindful of the time complexity of your code to prevent unexpected performance bottlenecks, especially with large datasets.
Working with `MutableSet` can present challenges. You need to ensure your objects have proper `__hash__` and `__eq__` implementations to avoid errors during set operations. Also, dynamic attribute creation in Python could lead to conflicts with the `MutableSet` structure, requiring careful management.
Circular dependencies between elements within your collection can lead to unexpected behavior, emphasizing the need for careful design and avoidance of such situations.
The `AttributeError` often stems from incorrect import statements or missing method implementations, underscoring the need to stay current with Python's changes and fully understand abstract base classes.
Understanding Python's MutableSet A Guide to Resolving AttributeError in Collections Module - Troubleshooting Steps for MutableSet-Related AttributeErrors
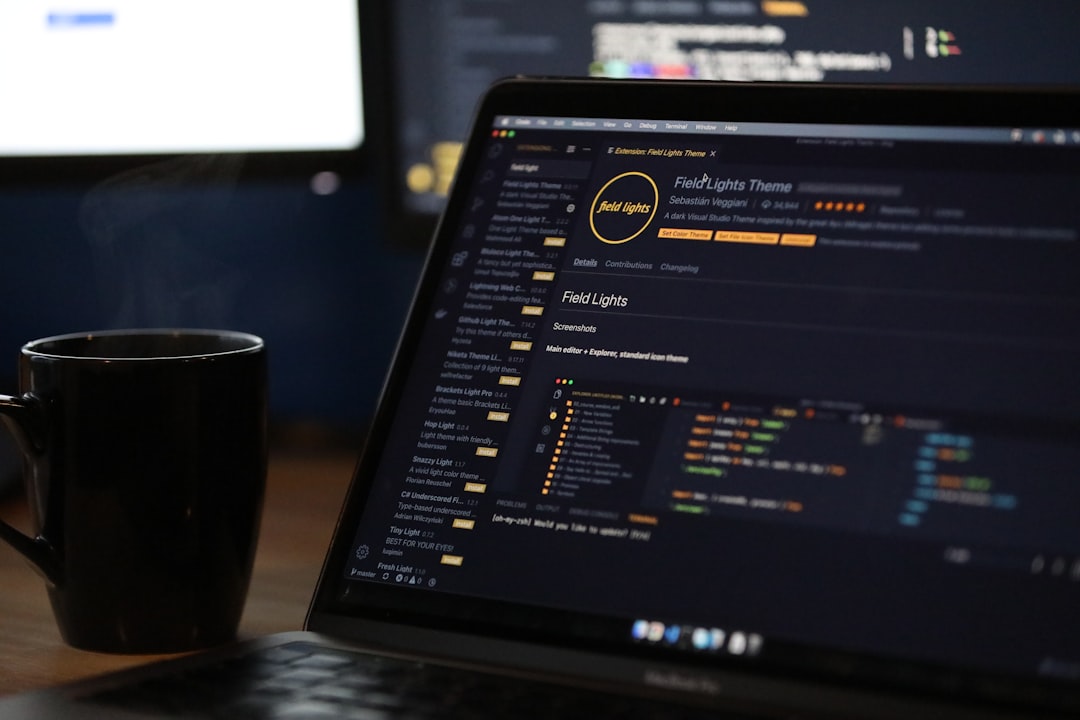
The "Troubleshooting Steps for MutableSet-Related AttributeErrors" section is crucial for anyone encountering errors related to Python's `MutableSet`. The core problem is that `MutableSet` has moved from the `collections` module to `collections.abc`, starting with Python 3.3. This means that if you are still trying to import `MutableSet` from the older location (`collections`), you'll run into an `AttributeError`. The fix is simple: change your import statement to `from collections.abc import MutableSet`. But this highlights a bigger issue – you need to stay on top of Python's changes.
This also brings up other key points:
* Make sure your code is compatible with your version of Python, as older code may not work properly with the new location of `MutableSet`.
* When creating custom `MutableSet` subclasses, ensure you have implemented all the required methods. Failing to do so will also trigger an `AttributeError`.
* Finally, always have robust error handling, especially when working with mutable data structures.
Delving into Python's `MutableSet` reveals some fascinating challenges, particularly with its transition from `collections` to `collections.abc` in Python 3.10. This change, though seemingly minor, underscores a significant shift in Python's development philosophy. It pushes us towards using abstract base classes, leading to cleaner and more structured code.
It's crucial to understand the core concept of `MutableSet` as an abstract base class. While it provides a framework for building customizable mutable sets, you can't directly instantiate it. Trying to do so will immediately trigger an `AttributeError`. This can be a surprise for new Python developers, and understanding this fundamental aspect is vital.
Another interesting observation is the difference in exception handling compared to standard Python sets. The `remove()` method in `MutableSet` throws a `KeyError` if the specified item is not present. This means custom implementations need to handle these exceptions carefully, unlike standard sets where these errors might be dealt with more subtly.
Let's talk about the impact of element types. `MutableSet` demands that all elements must be hashable. Using non-hashable types like lists or dictionaries can disrupt normal behavior, often leading to `AttributeError`. This emphasizes the importance of managing the types of data within these collections.
Furthermore, performance considerations come into play. Custom `MutableSet` implementations can differ in speed, depending on how the methods are defined. Poorly designed methods could lead to performance bottlenecks, particularly with larger datasets. It is vital to be aware of the time complexity involved.
Python's dynamic attribute creation can also be a source of trouble. It's possible for these dynamically created attributes to clash with the `MutableSet` structure, potentially causing `AttributeError`. This underscores the need for cautious development practices to avoid such conflicts.
Another point worth exploring is the importance of implementing appropriate `__hash__` and `__eq__` methods for custom objects used as elements in a `MutableSet`. Neglecting this can result in `AttributeError` during set operations, impacting expected behavior.
An unexpected cause of `AttributeError` arises from inconsistent environments. Running Python code in environments with mismatched packages or versions can lead to issues with `MutableSet`. Maintaining a consistent and well-organized environment is critical.
Finally, when overriding abstract methods in `MutableSet`, always remember to call the superclass methods to maintain correct behavior. Failing to do so can lead to unexpected `AttributeError`. This emphasizes the importance of understanding method inheritance and its impact.
These challenges highlight the complexities involved when working with `MutableSet`. While offering flexibility for customization, it demands careful attention to detail, meticulous error handling, and adherence to the underlying principles of abstract base classes.
Understanding Python's MutableSet A Guide to Resolving AttributeError in Collections Module - Future-Proofing Code When Working with Abstract Base Classes
Working with Abstract Base Classes (ABCs) in Python, especially when dealing with `MutableSet`, requires a focus on future-proofing your code. This means ensuring your code can handle changes in Python's specifications and remain functional. Using the `abc` module, you can define a consistent interface for your custom classes, forcing them to implement required methods. This not only helps prevent errors but also improves maintainability in the long run.
The `subclasshook` method is a powerful tool for adding flexibility to type-checking. This allows custom collections to work seamlessly with Python's standard types. By adhering to these design patterns, you can clarify the expected behavior of your classes and make your code more robust and adaptable to changes in Python's evolving specifications.
Python's abstract base classes (ABCs), like `MutableSet`, are becoming increasingly important for building robust and consistent code. ABCs serve as blueprints for classes, enforcing that subclasses implement certain methods, thus standardizing their behavior. This approach promotes code clarity and makes it easier to integrate different components.
With the introduction of generic type support in Python 3.10, `MutableSet` now allows developers to specify the types of elements stored in the set. This improves code safety and reliability by enabling static type checking, helping to avoid runtime errors caused by type mismatches.
However, this increased flexibility comes with a trade-off: the performance of custom `MutableSet` implementations can vary greatly, depending on the specific algorithms chosen. Careful consideration of time complexity is essential, especially when dealing with large datasets, to prevent performance bottlenecks.
Python's dynamic nature can also lead to unexpected behavior. The ability to create attributes dynamically can conflict with the structure of `MutableSet`. If custom attributes accidentally overwrite method names or expected behaviors, you're likely to encounter an `AttributeError`. This highlights the importance of coding carefully and avoiding potential conflicts with reserved names.
One significant difference between `MutableSet` and standard Python sets is the way exceptions are handled. The `remove()` method of `MutableSet` explicitly throws a `KeyError` if the element is not found. This contrasts with standard sets, where errors might be handled more subtly. This means custom `MutableSet` implementations require robust exception handling to ensure stability and predictability.
The concept of hashability is another important aspect of `MutableSet`. All elements must be hashable; using non-hashable types, like lists or dictionaries, directly will disrupt the set's normal operations. This reminds us that we must be mindful of data type compatibility when working with sets.
Another essential practice is to call superclass methods when overriding them in subclasses of `MutableSet`. Ignoring this principle can lead to inconsistent behavior and unexpected `AttributeError`. Understanding inheritance and its impact on method overriding is key to building robust custom classes.
Beyond individual features, the integration of `MutableSet` into the broader framework of `collections.abc` enhances the interplay between custom collections and more complex data structures. This interconnectedness expands the possibilities for developers and can lead to more sophisticated and modular designs.
The evolution of Python continues, and the shift of `MutableSet` to `collections.abc` is an example of this ongoing development. This underscores the need for developers to track language changes and stay updated, as code relying on outdated import paths can easily break.
Finally, as Python's community grows, adhering to best practices, including proper naming conventions and method signatures in custom `MutableSet` implementations, becomes increasingly vital. These coding standards not only make code more readable but also simplify collaboration among teams, ensuring a consistent and reliable approach.
More Posts from specswriter.com: