Implementing a Singleton UnitRegistry Pattern in Python Best Practices for Enterprise AI Applications
Implementing a Singleton UnitRegistry Pattern in Python Best Practices for Enterprise AI Applications - Standardizing Unit Registry Implementations Across Enterprise AI Applications
Maintaining a consistent approach to unit registries is vital for enterprise AI applications. This consistency is key for preventing errors, improving the flow of work, and making it easier for different parts of the system, and the teams that built them, to work together.
By organizing how units are handled, applications can avoid needless repetition and streamline data management, ultimately allowing different systems to seamlessly interact. Implementing patterns like the Singleton UnitRegistry, which ensures only one registry exists, simplifies managing units and conversions, especially in applications that rely heavily on math or science.
In large organizations, where various teams and modules are interconnected, standardized unit handling is crucial for overall system health and success. By focusing on best practices in implementing unit registries, AI applications can be built with a more unified and adaptable design. This avoids the potential chaos of having numerous, inconsistent approaches to units within the same system.
In the realm of enterprise AI, having a consistent way to handle units across different applications is crucial. Without standardization, it's easy for teams to use different units, causing delays as they try to figure out how they relate to each other. This focus on unit reconciliation diverts attention from the actual AI problems they're trying to solve.
We often find that a good chunk of errors in AI software projects come from inconsistent unit handling. This emphasizes how essential it is to establish a clear system for unit management. The Singleton UnitRegistry pattern can be beneficial by reducing redundant code, thereby making software easier to manage and maintain.
While converting units might seem simple, it can lead to rounding errors, especially in applications that heavily use data. This is particularly important in industries like finance and healthcare where tiny errors can have big consequences.
Surprisingly, unit testing for unit registries often gets overlooked. However, it's crucial for catching errors that may only show up under particular circumstances, making debugging much harder if they're not caught early.
Good communication across teams is vital in any project, and using a consistent unit registry can definitely help. It provides a common language for data scientists, engineers, and managers, leading to clearer requirements and improved workflows.
Using a common unit registry can also greatly improve the way different AI models work together. It forms a bridge for data exchange and processing, increasing overall efficiency.
The Singleton pattern helps prevent inconsistencies related to multiple copies of a unit registry by making sure all parts of an application use the same one. This simplifies debugging as it cuts down on the confusion of tracking which unit definition is being used.
It's not uncommon for developers to underestimate the importance of proper documentation for unit registry implementations. Without good documentation, it becomes a challenge when new team members join, slowing down development.
Finally, maintaining unit consistency is not just about accuracy. It's also crucial for meeting industry standards and regulations in fields like pharmaceuticals and automotive, where precision is non-negotiable. Proper unit management can be a significant factor in ensuring a system meets those requirements.
Implementing a Singleton UnitRegistry Pattern in Python Best Practices for Enterprise AI Applications - Thread Safe Singleton Design for High Volume Data Processing
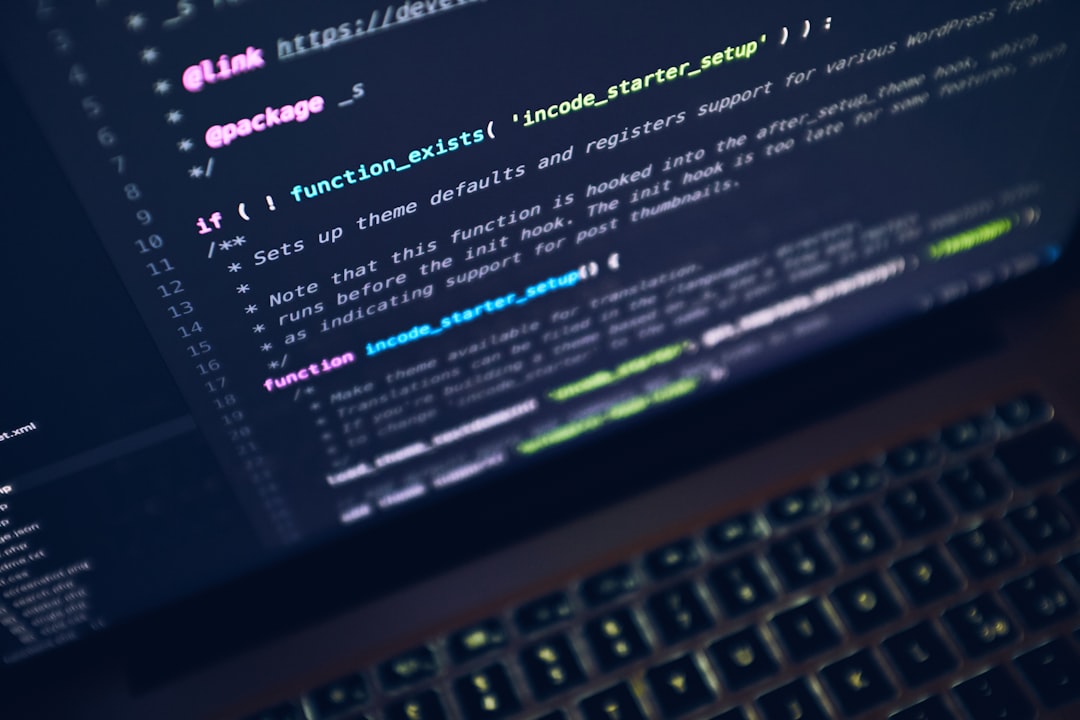
When dealing with large amounts of data processed concurrently, having a thread-safe singleton design is crucial for efficiency and stability. The Singleton pattern helps prevent multiple instances of a class from being created, which can cause issues like inconsistent states and errors due to multiple threads trying to access and modify the same resources at the same time (race conditions). Methods like the Initialization-on-demand holder idiom and double-checked locking can be used to create thread-safe singletons with minimal performance penalties. However, it's important to be mindful of common mistakes like inadequate synchronization, which could still lead to issues in a multithreaded environment. The value of a well-implemented thread-safe singleton lies in its ability to manage shared resources (like database connections) smoothly, leading to improvements in application performance and reliability—especially in demanding enterprise AI applications.
The Singleton pattern, while useful for ensuring a single instance of a class, presents unique challenges when dealing with high-volume data processing. One big issue is that if you don't handle concurrency correctly, even a Singleton can create performance problems because multiple threads might try to access the same resource at once. This can create a lot of waiting and slow everything down.
There's also the issue of memory. Thread-safe Singletons often need extra structures for keeping track of things like locks, which can eat up valuable memory, particularly if you're already working in a limited resource environment. It's a constant trade-off: you want it to be safe, but you don't want it to bog everything down.
Finding the right balance between performance and safety can be a challenge, too. You can't just lock everything down all the time because that could slow things to a crawl, but if you're too relaxed, you risk ending up with multiple threads changing the same data at the same time - creating errors you don't want. And you have to think about potential deadlocks where threads are waiting for each other forever, effectively halting the system.
When you use lazy initialization, where the Singleton isn't created until it's actually needed, you can get unexpected behavior under heavy load. Threads might find that the resource they need isn't ready yet, which can cause problems in the flow of the data processing.
Testing is also a real challenge in this context. Ensuring that the Singleton works correctly when many things are going on at once requires really sophisticated testing methods, which can be a lot of work.
Singletons have the potential to cause issues with global state, creating complex, difficult-to-debug situations when multiple modules interact. This is a concern particularly for complex AI systems. What might seem like a good solution for one part can inadvertently affect another without anyone noticing.
It can be hard to adapt a system that uses a Singleton. As your application needs change, you might need to change your approach to processing data. A Singleton, though, inherently has limitations because it's designed to be a single, centralized point.
High-volume environments might end up with unpredictable usage patterns for the Singleton. You might find that the Singleton becomes the main chokepoint of the system - meaning if that part fails, everything fails. The reliability of your system would be heavily dependent on this one element.
Lastly, there are compliance and security considerations. If your Singleton holds sensitive information and anyone can access it, you could expose private data, requiring extra safeguards, which in turn makes everything more complex.
Implementing a Singleton UnitRegistry Pattern in Python Best Practices for Enterprise AI Applications - Memory Management and Resource Allocation in Python Unit Registry
When implementing a Singleton UnitRegistry in Python for enterprise AI applications, paying close attention to memory management and how resources are used is crucial. Python's memory management is largely automatic, relying on a dedicated heap where all data lives. The Python memory manager handles this heap and uses a method called reference counting to efficiently manage memory allocation. This means that memory is automatically released when no part of the program is referencing it any more.
However, in situations with high volumes of data processed concurrently, using a thread-safe Singleton design can introduce new challenges. The methods used to make a Singleton thread-safe, like locks and other synchronization mechanisms, can add overhead and require more memory. This can, in turn, create performance bottlenecks, especially if you're already dealing with a limited amount of system resources.
Therefore, you have to carefully consider these factors when building an application that needs to handle a lot of data at the same time while also using a Singleton UnitRegistry. Balancing efficient resource usage with system reliability is key, especially for enterprise AI applications that depend on the consistent and predictable handling of units to function correctly. Understanding these tradeoffs is important for developing well-performing, stable, and robust enterprise AI systems.
When we're dealing with thread-safe singleton designs within a Python unit registry, a few interesting memory-related things pop up. First, the synchronization mechanisms needed to ensure thread safety—like locks or semaphores—can use up more memory than we might expect. This becomes especially important if our system is already strapped for memory.
Python's usual memory management, which uses reference counting, can also be a bit of a challenge for singletons. The bookkeeping involved in tracking references can cause a bit of performance slowdown and impact how efficiently memory is allocated, potentially impacting the garbage collection process.
Things get a bit more complex with the Global Interpreter Lock (GIL) in CPython. Even with our thread-safe designs, true parallelism is still limited by the GIL, meaning only one thread can run Python bytecode at a time. This can affect how well a singleton performs in high-volume data processing applications, as it can create performance bottlenecks that impact responsiveness.
We also need to consider resource contention. When multiple threads are fighting for access to the same singleton instance, it can create delays and performance slowdowns, especially when things get busy. This isn't necessarily what we want from a design meant to increase efficiency.
There are also some quirks with lazy initialization in singletons. If we don't handle things carefully, multiple threads could try to create the singleton at the same time, potentially leading to unintended consequences—multiple instances when we only want one.
Overuse of locking can potentially lead to thread starvation, where some threads get left out in the cold, unable to access the singleton. In real-time systems where we care about speed and response, this is a problem.
The memory management of a singleton unit registry is also pretty tricky. We need to carefully track how it's being used or risk memory leaks. If memory is allocated but isn't freed up later on, it can lead to a situation where we have more memory taken up than we need.
Singletons that handle a lot of tasks in high-volume applications can become a bottleneck. If they're being accessed far more often than expected, they can restrict throughput. This might require extra steps to manage it better, like caching or load balancing, so the workload is handled more smoothly.
Another aspect is compatibility with other design patterns. Adding a singleton to the mix can make it a bit harder to integrate things like dependency injection or service locators. They're designed to create instances when needed, which goes against the fixed-instance idea that's central to a singleton.
Finally, singletons can create some debugging challenges. When we have a shared global resource, changes made from different parts of the application can be hard to trace, leading to problems that only show up in specific circumstances. These hard-to-pinpoint bugs can make maintenance a bit more difficult.
It's all a bit of a balancing act to make sure singletons work effectively while managing these potential challenges, especially within the context of enterprise AI applications where there are often constraints on memory and performance.
Implementing a Singleton UnitRegistry Pattern in Python Best Practices for Enterprise AI Applications - Error Handling and Custom Exception Strategies for Singleton Patterns
When implementing a Singleton pattern, particularly for a UnitRegistry in enterprise AI, you encounter a unique set of error scenarios. The Singleton's nature, guaranteeing a single instance, means that errors related to instance creation or access become crucial to handle properly. To improve robustness, we often design custom exception types that clearly indicate the specific error encountered. This could involve separate exceptions for scenarios like failed initialization or multiple access attempts.
Python offers the `from e` syntax, which is a good practice when raising a new exception based on an existing one. This maintains the chain of exceptions, which is handy for debugging complex error situations as it preserves the original traceback. Additionally, we can leverage Python's structured exception handling—the `try`, `except`, and `finally` blocks—to create more graceful error handling.
It's important to follow good exception handling guidelines here. This means being specific about the types of errors you're catching and providing descriptive messages that make it easier to understand and resolve issues. Including logging of errors is also important for debugging. You should only use exceptions for exceptional cases, not as a means for typical control flow within the application.
The benefits of improved exception handling within Singleton patterns are numerous in the context of enterprise AI systems. The ability to anticipate and manage potential errors improves the overall stability and reliability of the system. When these systems are dealing with important data, particularly for applications in areas like finance or healthcare, precise error handling is essential. It allows for more robust and maintainable applications, especially critical for enterprise applications that may have complex interactions and dependencies.
When employing the Singleton pattern, particularly in a multithreaded context like our UnitRegistry example, a few interesting issues crop up with error handling. If one thread encounters an error and doesn't handle it gracefully, the problem can spread to other threads trying to use the same Singleton, leading to a larger failure. This emphasizes the importance of designing error recovery and handling mechanisms that can isolate and manage these errors without causing chaos across the whole system.
Lazy initialization, a common technique where the Singleton isn't created until needed, can introduce timing problems in high-concurrency settings. If multiple threads attempt to access the Singleton simultaneously before it's been properly initialized, this can result in strange application behavior—potentially even creating multiple instances when we only intended to have one.
Another challenge with Singletons comes from managing access in a threaded environment. Thread-safety, often accomplished with locking mechanisms, can create its own bottleneck. If lots of threads try to access the same resource at once, it can create a logjam, negating the benefits of a single instance and possibly slowing things down.
Furthermore, synchronization methods, like mutexes or semaphores, come with a memory cost. This becomes more critical in resource-limited situations. Every bit of memory counts, and adding extra bits simply to maintain thread safety might not be a worthwhile trade-off in some cases.
Python's Global Interpreter Lock (GIL) presents an additional challenge in multi-threaded environments. The GIL restricts true parallelism, potentially limiting performance gains a Singleton might otherwise offer. So, while the Singleton pattern inherently avoids certain problems by only allowing a single instance, this advantage can be somewhat eroded by the presence of the GIL.
Managing a Singleton's lifespan can also be a source of headaches, especially when it comes to memory management. We must ensure that it's set up properly, used correctly, and disposed of gracefully without causing memory leaks, especially in applications that run for a long time.
To better deal with these nuances, we can define our own exception types, customized for the Singleton pattern. This lets us handle specific problems related to shared resource access or concurrent modifications in a more elegant and targeted way. Standard exception handling may not be sufficient to effectively manage situations arising from the Singleton's behavior.
The global nature of a Singleton also creates some interesting debugging challenges. When multiple threads are manipulating the same data, tracing down the origin of a problem can get quite convoluted. Debugging efforts are often made more difficult in these situations, so proactively building systems to catch potential problems early can be essential.
Interestingly, a Singleton's performance varies significantly based on how many threads are using it. In low-concurrency settings, it typically performs quite well. However, as the number of threads grows, contention and the overhead of thread safety start to negatively impact performance.
And lastly, testing Singletons can be a challenge because their global state might influence how individual tests run. Tests might unexpectedly affect each other, requiring careful design and the potential use of mocking mechanisms to isolate elements from one another. This further complicates the already intricate task of testing software.
These points reveal that using Singleton patterns, while beneficial for resource management, requires careful consideration of potential problems related to error handling, concurrency, performance, and testing. This is especially true for enterprise-scale AI applications where system reliability is critical.
Implementing a Singleton UnitRegistry Pattern in Python Best Practices for Enterprise AI Applications - Integration Testing Methods for Unit Registry Components
When implementing a Singleton UnitRegistry, integration testing becomes crucial for verifying how different parts of an AI application work together. Unlike unit tests that focus on individual components, integration tests evaluate how modules interact, especially at their connection points. Techniques like mocking and patching play a crucial role in integration testing. By simulating dependencies, these methods allow for a deep examination of interactions without the need to involve real-world systems. This helps uncover flaws in how components communicate, ensuring the Singleton UnitRegistry's behavior remains consistent when multiple threads are involved. It's vital that testing methodologies be comprehensive and robust to guarantee the reliability of applications heavily reliant on standardized units. These tests are critical to ensure that the singleton design remains thread-safe and functional within the larger system. Without proper integration tests, subtle issues may only arise in complex real-world situations, potentially leading to unexpected results or failures. Given the vital role a Singleton UnitRegistry plays in standardizing data handling within enterprise AI applications, it's essential that these integration tests are performed regularly and thoroughly as new features are added or systems are modified.
1. When doing integration testing for unit registry parts, we often find hidden connections that weren't obvious during unit testing. This means we need a plan that checks not only how the registry itself works but also how it interacts with other parts of the system. This helps us find potential problems early on, before they cause bigger issues.
2. A common mistake when doing integration testing with Singleton unit registries is thinking that their thread safety is enough for tests with multiple threads running at once. Under heavy use, issues related to multiple threads trying to do things at the same time can still happen, which can give us misleading test results and hide real bugs.
3. It's interesting that integration tests can also act as a kind of documentation. They don't just check if the code works, they also show us how the unit registry is supposed to behave in different situations. This is often more useful than regular documentation on its own.
4. The way Singletons use memory, along with the extra memory integration tests need, can sometimes lead to the system running out of resources during testing. If we don't think about how resources are used when we design our tests, this can affect the results in ways we might not expect.
5. Using tools to fake dependencies during integration testing can be counterproductive if we aren't careful. If we rely too much on fakes, we might not fully understand how the Singleton unit registry works with real data and other components. This can reduce the effectiveness of our tests.
6. It's really important to make sure our integration tests match how the system is actually used in the real world. Tests that aren't like what happens in a production environment might miss important problems. For unit registries, this means simulating how data flows and how many threads access the registry in a typical enterprise application.
7. The order in which different parts of the system are set up can have a big impact on how fast integration tests that use Singleton registries run. If tests don't consider the order in which components are set up, it can lead to confusing results and false negatives during testing.
8. Surprisingly, performance testing is often skipped in integration test sets. Since the Singleton pattern keeps everything in one place, it's important to measure how well it handles different loads to see how it behaves in real-world situations.
9. Integration tests also show us how important it is to have a good versioning system for unit registries. If we change how units are defined or how conversions are done, it can have a ripple effect. Integration tests are better than isolated unit tests at showing these ripple effects.
10. Lastly, if we don't have good error handling in both the Singleton code and in our integration tests, it can lead to system stability problems later on. Tests shouldn't just check if things work as expected, they also need to make sure the system is robust enough to handle unusual and error-prone scenarios that could disrupt the service.
Implementing a Singleton UnitRegistry Pattern in Python Best Practices for Enterprise AI Applications - Automated Deployment Pipelines for Unit Registry Updates
Automating the deployment process for unit registry updates is crucial in enterprise AI applications. These automated pipelines help streamline the delivery of new registry features and bug fixes, ensuring seamless integration with the rest of the system. This includes efficiently handling changes to unit definitions or conversion methods. Tools such as Jenkins or GitHub Actions can be used to build pipelines that manage the release and distribution of updates, supporting consistent use of a Singleton UnitRegistry across the various parts of an AI application.
However, the implementation of these automated deployment pipelines requires careful planning and maintenance. Poorly designed pipelines can introduce new vulnerabilities and bottlenecks, potentially undermining the core functionality of the unit registry. Clear, well-defined, and easily maintained deployment procedures are thus essential for ensuring the long-term health and reliability of complex AI environments. Failing to create a robust deployment pipeline can mean inconsistent unit handling or failures when new versions of the registry are introduced. Ideally, this is designed to help developers get new features and fixes into production with fewer problems and without disrupting the applications using it.
Pint, a Python library for handling physical quantities, necessitates a `UnitRegistry` object for defining and converting units. To avoid the potential problems of multiple `UnitRegistry` instances, it's best practice to initialize it in a central location like the `__init__.py` file of your package. This avoids inconsistencies where units from different registries aren't interchangeable, causing confusion and errors.
Modern software development leans heavily on automated deployment pipelines. These pipelines streamline the process of releasing new features and updates, helping organizations keep pace with the demands of their applications. Crafting a robust automated pipeline requires careful thought, selecting the right tools, and continually improving based on user feedback and experience.
Jenkins, a popular tool for automating tasks, can be a useful piece in the puzzle of deploying updates. It handles processes like building and packaging Python applications, enabling seamless transitions between testing and production environments. Ideally, it should be set up to scale efficiently, especially for larger projects, with features like distributed builds and a master-agent architecture to handle the workload effectively.
Tools like GitHub Actions offer a streamlined approach to establishing a continuous integration (CI) pipeline. You can create a repository, define the project's requirements, and let the tool orchestrate the build process.
When building deployment processes, it's insightful to draw upon ideas from the Twelve-Factor App methodology. It promotes principles like "API First" and "Port Binding" which can reduce dependencies and silos between teams, potentially leading to more streamlined and effective deployment procedures.
It's crucial to create automated deployment procedures that are both well-documented and easy to follow. Clarity and maintainability are essential, as the procedures will likely need adjustments over time as the software evolves. Well-designed pipelines also make it easier for new engineers to contribute to the development and deployment of future versions of the software. Keeping the deployment procedures readable and accessible makes it easier to share knowledge and responsibility for system upgrades.
However, it's worth noting that automated deployment isn't a magical solution. Without proper oversight, pipelines can become outdated, and if not maintained effectively, could even become sources of new problems. This requires ongoing evaluation and adjustments to make sure they remain current and reliable. Moreover, while these tools simplify the process of deploying updates, they need to be understood and managed effectively to avoid unintended consequences.
One common challenge with pipelines is a lack of good communication between teams. In a large organization, it's important to create processes where updates are tracked and visibility is ensured. Clear and accessible updates on deployments are vital for building a cohesive and collaborative environment. The tools should be used to encourage this cooperation, rather than simply automating the process in a vacuum. Clear communication on changes allows developers and stakeholders to easily understand what's happening and ensures updates are properly vetted, leading to improved quality and stability.
Finally, it's a reminder that the choice of automated deployment tools should fit the organization and project. While some tools and workflows may be better known, it's essential to find the right match that is adaptable and effective for a particular context. Blindly applying a popular approach without considering the nuances of the application could result in inefficiencies or difficulties.
More Posts from specswriter.com: